Allows third-party developers to authorize using OAuth 2.0
We use the industry-standard protocol for authorization. OAuth 2.0 provides access to our public APIs with the help of exchange of an authorization token for an access token. For detailed specification, check out the OAuth 2.0 Authorization Framework.
Here is a quick summary of the Authorization Code Flow:
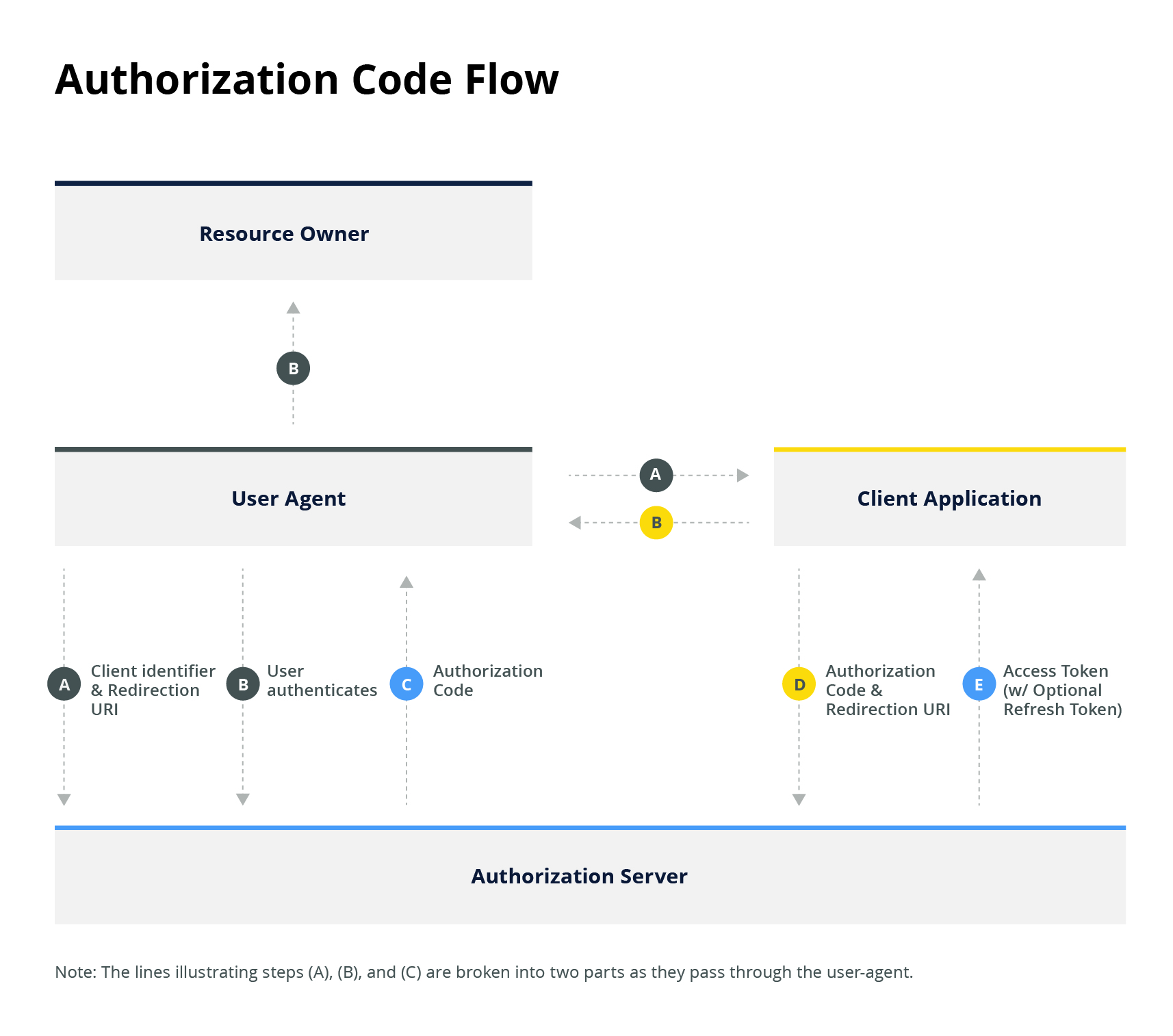
After a successful setup of a developer account with Motive's support staff, a developer can log in to the Motive developer portal. The developer needs to register an application on this portal. After registering your app, you will receive a client ID
and a client secret
. Client ID is considered public information and is used to build the login URLs. The client secret must be kept confidential.
To complete the authorization flow, you need to display a button that starts the connection process /oauth/authorize
and a redirect page that would redirect the user back after connecting the application. Full URL could be like this:
https://api.gomotive.com/oauth/authorize?client_id=<uid of application>&redirect_uri=<redirect_uri of application>&response_type=code&scope=<all scopes mentioned in application definition>
Argument | Value | Description | Required |
---|---|---|---|
client_id | uid of application | The client ID you received when you first created the application | Yes |
redirect_uri | Redirect URI of the application | Indicates the URI to return the user to after authorization is complete. | Yes |
response_type | code | Indicates that your server expects to receive an authorization code. | Yes |
scope | Scopes mentioned in the application definition: companies.read users.read users.manage vehicles.read vehicles.manage messages.read messages.manage fuel_purchases.read fuel_purchases.manage groups.read groups.manage eld_devices.read inspection_reports.read fault_codes.read driver_performance_events.read hos_logs.available_time hos_logs.hours_of_service hos_logs.hos_violation hos_logs.logs locations.vehicle_locations_list locations.vehicle_locations_single locations.driver_locations ifta_reports.trips ifta_reports.summary utilization.driver_utilization utilization.vehicle_utilization utilization.idle_events documents.read dispatches.read dispatches.manage dispatch_locations.read dispatch_locations.manage forms.read form_entries.read freight_visibility_vehicle_locations.read freight_visibility_vehicle_locations.manage freight_visibility_asset_locations.read freight_visibility_asset_locations.manage | One or more scope values indicating which parts of the user's account you wish to access. | Yes |
After clicking the button, users are taken to a page like the one below to review the requested permissions and link to their account:
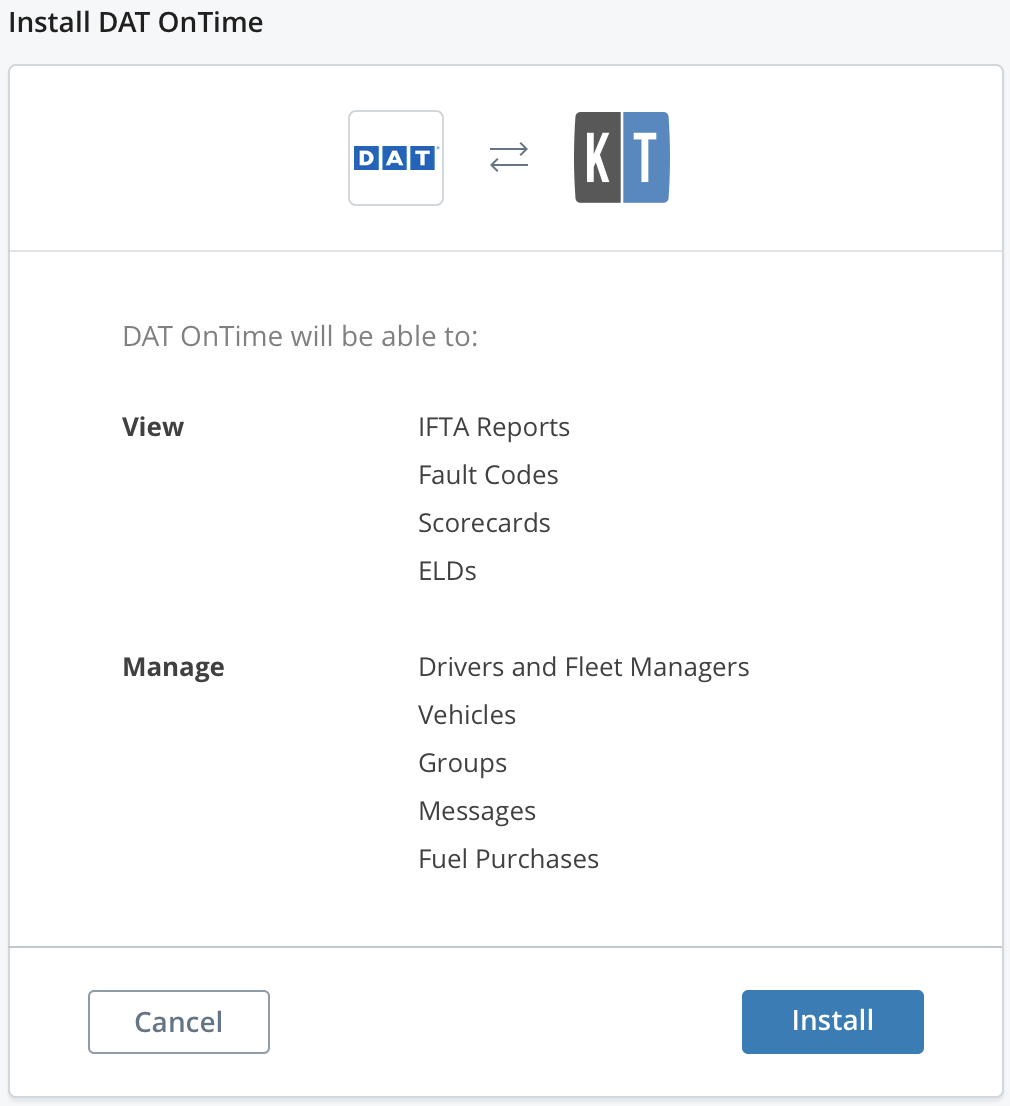
Request to authorize the application to link to KeepTruckin
When the user clicks on Allow, the page will be redirected to the developer's provided redirect URI. The server exchanges the auth code for an access token by hitting /token
endpoint.
Note: The auth code time to live is 10 min. If the auth code is not exchanged for an access token, the auth code will expire, and the customer would need to reauthorize the app to generate a new auth code.
curl -X POST 'https://api.gomotive.com/oauth/token' -H "Content-Type: application/x-www-form-urlencoded" -d 'grant_type=authorization_code&code=<code returned after resource owner authorization>&redirect_uri=<redirect url specified in developer app>&client_id=<uid of application>&client_secret=<secret of applicatio>'
require 'uri'
require 'net/http'
require 'json'
url = URI('https://api.gomotive.com/oauth/token')
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request['Content-Type'] = 'application/x-www-form-urlencoded'
params = {
:grant_type => 'authorization_code',
:code => '<code returned after resource owner authorization>',
:redirect_uri => '<redirect url specified in developer app>',
:client_id => '<uid of application>',
:client_secret => '<secret of application>',
}
request.body = params.to_json
response = http.request(request)
puts response.read_body
The server replies back with an access token and expiration time.
{
"access_token": "de6780bc506a0446309bd9362820ba8aed28aa506c71eedbe1c5c4f9dd350e54",
"token_type": "Bearer",
"expires_in": 7200,
"refresh_token": "8257e65c97202ed1726cf9571600918f3bffb2544b26e00a61df9897668c33a1"
}
Once an access token is expired, the developer can simply get a new access token using the refresh token that is returned in initial response like following.
curl -X POST 'https://api.gomotive.com/oauth/token' -H "Content-Type: application/x-www-form-urlencoded" -d 'grant_type=refresh_token&refresh_token=<refresh token returned with access token>&redirect_uri=<redirect url specified in developer app>&client_id=<uuid of application>&client_secret=<secret of applicatio>'
require 'uri'
require 'net/http'
require 'json'
url = URI('https://api.gomotive.com/oauth/token')
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request['Content-Type'] = 'application/x-www-form-urlencoded'
params = {
:grant_type => 'refresh_token',
:refresh_token => '<refresh_token returned with access_token>',
:redirect_uri => '<redirect url specified in developer app>',
:client_id => '<uid of application>',
:client_secret => '<secret of applicatio>',
}
request.body = params.to_json
response = http.request(request)
puts response.read_body
Once the OAuth access token is obtained, you can start making requests to the API. A bearer token is required to make requests.
Header Name | Value |
---|---|
Authorization | Bearer |
Sample API call to /v1/users
endpoint.
curl --request GET 'https://api.gomotive.com/v1/users' -H 'Authorization: Bearer <OAUTH_TOKEN>'
require 'uri'
require 'net/http'
url = URI('https://api.gomotive.com/v1/users')
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Get.new(url)
request['Authorization'] = 'Bearer <OAUTH_TOKEN>'
response = http.request(request)
puts response.read_body
var request = require("request");
var options = { method: 'GET', url: 'https://api.gomotive.com/v1/users', headers: {'Authorization': 'Bearer <OAUTH_TOKEN>'} };
request(options, function (error, response, body) {
if (error) throw new Error(error);
console.log(body);
});